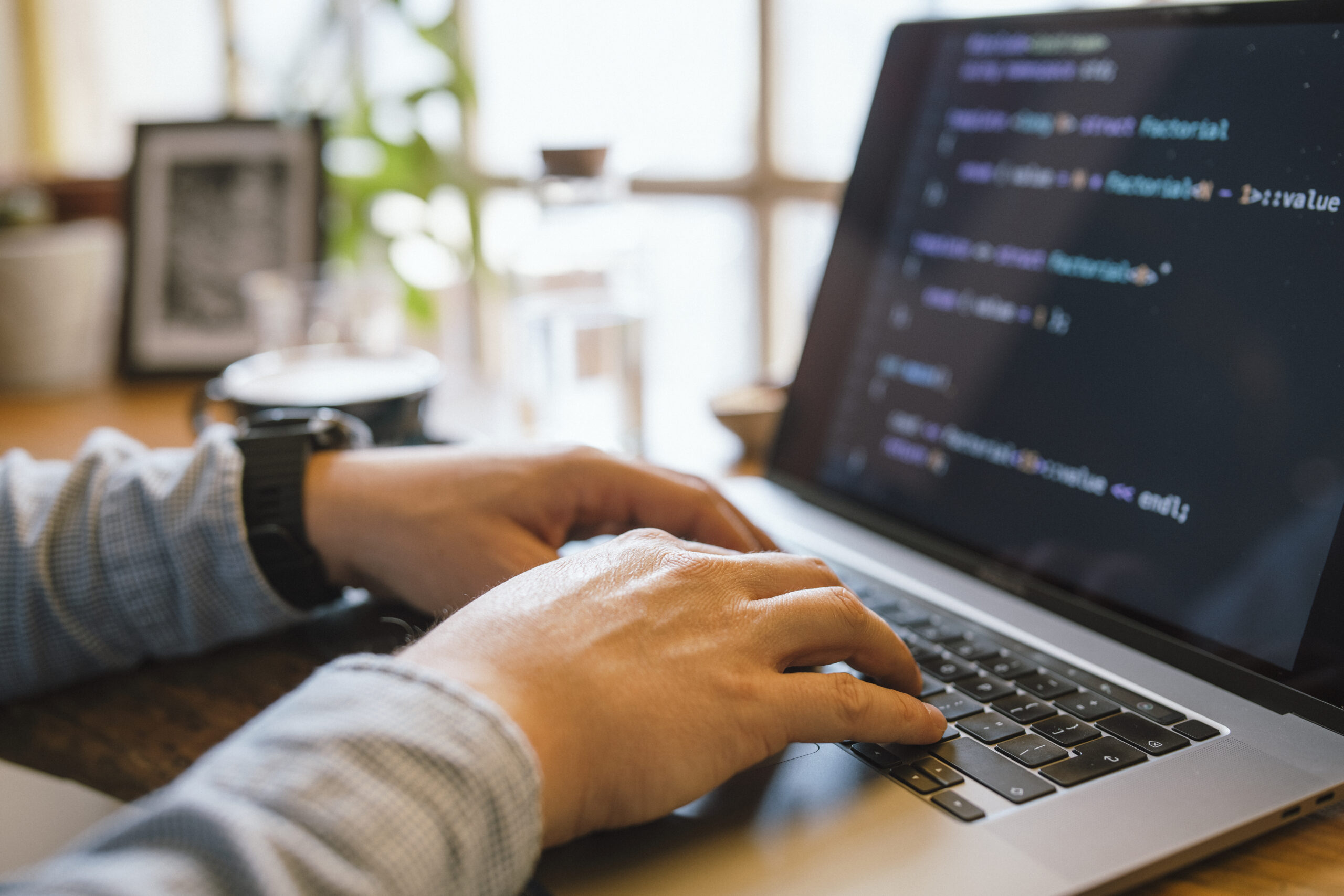
Debugging is one of the most vital — yet frequently missed — abilities within a developer’s toolkit. It's actually not almost correcting damaged code; it’s about being familiar with how and why things go Incorrect, and Understanding to Consider methodically to resolve troubles proficiently. No matter if you are a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of aggravation and significantly enhance your productivity. Listed here are several techniques to aid developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
On the list of fastest approaches developers can elevate their debugging expertise is by mastering the resources they use each day. Whilst creating code is 1 Portion of advancement, figuring out tips on how to connect with it properly in the course of execution is equally vital. Present day advancement environments arrive Geared up with highly effective debugging capabilities — but quite a few developers only scratch the surface of what these instruments can do.
Choose, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, action by means of code line by line, and even modify code about the fly. When utilized effectively, they Allow you to notice precisely how your code behaves through execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-close developers. They assist you to inspect the DOM, keep track of community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch disheartening UI concerns into workable responsibilities.
For backend or program-amount developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management over jogging procedures and memory management. Mastering these applications might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, come to be comfy with Edition Management devices like Git to understand code background, locate the precise minute bugs ended up launched, and isolate problematic alterations.
In the long run, mastering your applications means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your progress ecosystem so that when problems arise, you’re not misplaced at midnight. The better you realize your resources, the more time you are able to invest solving the particular problem rather than fumbling through the procedure.
Reproduce the condition
One of the more significant — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, builders need to have to create a constant atmosphere or scenario where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a match of possibility, frequently bringing about squandered time and fragile code modifications.
The initial step in reproducing a challenge is gathering just as much context as you can. Inquire questions like: What steps led to The difficulty? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it gets to isolate the exact conditions underneath which the bug occurs.
As soon as you’ve collected plenty of info, seek to recreate the challenge in your neighborhood surroundings. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge conditions or state transitions included. These tests not simply help expose the trouble but will also stop regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on certain working devices, browsers, or less than specific configurations. Employing instruments like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But as soon as you can continuously recreate the bug, you might be already halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment additional proficiently, exam opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary grievance right into a concrete obstacle — Which’s wherever builders thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most valuable clues a developer has when something goes wrong. Rather than looking at them as discouraging interruptions, developers must discover to treat mistake messages as direct communications in the system. They normally inform you what exactly happened, where it transpired, and often even why it happened — if you know the way to interpret them.
Start out by reading through the message diligently and in complete. Lots of developers, especially when underneath time strain, glance at the 1st line and quickly commence making assumptions. But further in the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like google — browse and recognize them first.
Split the mistake down into areas. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and position you towards the accountable code.
It’s also handy to comprehend the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can significantly hasten your debugging process.
Some mistakes are obscure or generic, As well as in These situations, it’s very important to examine the context during which the mistake happened. Verify connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach begins with realizing what to log and at what degree. Typical logging ranges include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic info throughout improvement, INFO for general situations (like prosperous start off-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Give attention to important occasions, point out alterations, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, for instance timestamps, ask for IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a nicely-imagined-out logging solution, you'll be able to decrease the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers need to technique the procedure similar to a detective solving a mystery. This attitude will help stop working advanced challenges into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect as much relevant information as you are able to without jumping to conclusions. Use logs, test cases, and user reviews to piece with each other a clear photo of what’s taking place.
Up coming, kind hypotheses. Request your self: What might be creating this behavior? Have any variations a short while ago been designed on the codebase? Has this concern occurred before less than very similar conditions? The aim would be to slender down options and discover possible culprits.
Then, test your theories systematically. Seek to recreate the challenge within a managed natural environment. Should you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, ask your code issues and Allow the effects direct you nearer to the truth.
Pay near interest to compact specifics. Bugs often cover within the the very least expected destinations—like a lacking semicolon, an off-by-1 mistake, or perhaps a race affliction. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help save time for future troubles and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become more effective at uncovering hidden difficulties in elaborate methods.
Publish Assessments
Crafting tests is one of the most effective approaches to transform your debugging competencies and overall improvement effectiveness. Exams not simply enable capture bugs early but will also function a security Web that provides you self confidence when generating improvements towards your codebase. A perfectly-tested software is much easier to debug mainly because it allows you to pinpoint exactly where and when a problem occurs.
Start with unit checks, which focus on individual capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain piece of logic is Functioning as anticipated. When a test fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Device checks are Specially beneficial for catching regression bugs—problems that reappear following Beforehand staying mounted.
Up coming, integrate integration assessments and stop-to-finish checks into your workflow. These enable be certain that numerous parts of your software function together efficiently. They’re specifically useful for catching bugs that come about in sophisticated systems with many elements or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect effectively, you need to grasp its inputs, expected outputs, and edge situations. This level of comprehension naturally qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails consistently, it is possible to focus on fixing the bug and look at your exam pass when The problem is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—watching your display screen for hrs, hoping Answer right after Resolution. But Among the most underrated debugging applications is solely stepping away. Using breaks will help you reset your head, lower annoyance, and infrequently see The problem from the new point of view.
When you are way too near to the code for also extended, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. During this point out, your Mind gets considerably less productive at difficulty-solving. A brief wander, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your focus. Lots of builders report obtaining the basis of an issue when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support stop burnout, especially all through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you before.
When you’re stuck, a very good rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weakness—it’s a wise strategy. It provides your Mind space to breathe, enhances your standpoint, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of website solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious for those who make an effort to reflect and evaluate what went Mistaken.
Start out by inquiring you a few key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Establish much better coding behaviors transferring ahead.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you can proactively stay away from.
In group environments, sharing what you've acquired from the bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential areas of your improvement journey. In spite of everything, a number of the most effective developers are usually not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Every bug you deal with adds a whole new layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, extra capable developer on account of it.
Summary
Improving your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.